kennt jemand ne Methode, mit welcher ich von einem Punkt aus eine bestimmte Entfernung in eine bestimmte Richtung gehe und die mir die Koordinaten von diesem neuen Punkt berechnet?
Erstelle dir eine Vektor Klasse. Dann brauchst du nur einen Richtungsvektor mit dem Entsprechenden Winkel erzeugen. Dieser wird dann mit der geforderten Länge multipliziert und schließlich zum Startpunkt addiert.
Hier eine Demo wie man das realisieren könnte.
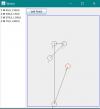
[CODE lang="java" title="TestVector2D" highlight="7"]import javax.swing.JFrame;
public class TestVector2D {
public static void main(String[] args) {
JFrame frame = new JFrame("Vector");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new VectorPanel(500, 500));
frame.pack();
frame.setResizable(false);
frame.setVisible(true);
}
}[/CODE]
[CODE lang="java" highlight="48-51"]import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Iterator;
import java.util.Vector;
import javax.swing.DefaultListModel;
import javax.swing.JButton;
import javax.swing.JList;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
@SuppressWarnings("serial")
public class VectorPanel extends JPanel {
private static JTextField[] input = { new JTextField(new MyFloatDocument(), "", 1), new JTextField() };
private static String[] label = { "Winkel", "Länge" };
public final static int ANGEL = 0;
public final static int LENGHT = 1;
private JButton btnAdd = new JButton("add Point");
private DefaultListModel<String> model = new DefaultListModel<String>();
private JList<String> list = new JList<String>(model);
private Vector<Vector2D> path = new Vector<Vector2D>();
private Rectangle bounds;
public VectorPanel(int width, int height) {
setLayout(null);
add(btnAdd);
add(list);
updateSize(width, height);
path.add(new Vector2D(width / 2, height / 2));
btnAdd.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int ok = JOptionPane.showConfirmDialog(null, getObjects(), "Positionswerte eingeben",
JOptionPane.OK_OPTION);
if (ok == JOptionPane.OK_OPTION)
addPos();
}
private void addPos() {
double angle = Double.parseDouble(input[ANGEL].getText());
double lenght = Double.parseDouble(input[LENGHT].getText());
Vector2D pos = path.lastElement();
Vector2D direction = new Vector2D(angle);
direction = direction.multiply(lenght);
pos = pos.add(direction);
if (!pos.isInside(bounds)) {
JOptionPane.showMessageDialog(null, pos + " is outside of allowed bounds!");
return;
}
int size = path.size();
model.add(size - 1, String.format("%d W %.1f, L%.1f", size, angle, lenght));
path.add(pos);
repaint();
}
});
}
public void drawPoint(Graphics g, Vector2D point, int num) {
int sizeP = g.getFont().getSize() * 2;
int x = point.intX() - sizeP / 2;
int y = point.intY() - sizeP / 2;
g.drawOval(x, y, sizeP, sizeP);
g.setColor(Color.GREEN.darker());
g.drawString(num + "", x, y + 3 * sizeP / 2);
}
private static Object[] getObjects() {
Object[] fields = new Object[label.length * 2];
int cnt = 0;
for (int i = 0; i < fields.length - 1; i += 2) {
fields
= label[cnt];
fields[i + 1] = input[cnt];
cnt++;
}
return fields;
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.BLUE);
g.drawRect(bounds.x, bounds.y, bounds.width, bounds.height);
Iterator<Vector2D> it = path.iterator();
Vector2D start = it.next();
int cnt = 1;
while (it.hasNext()) {
Vector2D end = it.next();
g.setColor(Color.DARK_GRAY);
g.drawLine(start.intX(), start.intY(), end.intX(), end.intY());
drawPoint(g, start, cnt++);
start = end;
}
g.setColor(Color.RED);
drawPoint(g, start, cnt);
}
private void updateSize(int width, int height) {
setPreferredSize(new Dimension(width, height));
int frame = 5;
int listWidth = (width - 2 * frame) / 4;
int listHeight = height - 2 * frame;
int btnHeight = 30;
list.setBounds(frame, frame, listWidth, listHeight);
btnAdd.setBounds(frame * 2 + listWidth, frame, 100, btnHeight);
bounds = new Rectangle(listWidth + 3 * frame, 2 * frame + btnHeight, width - listWidth - 4 * frame,
height - 4 * frame - btnHeight);
}
}[/CODE]
[CODE lang="java" title="Vector2D"]import java.awt.Point;
import java.awt.Rectangle;
public class Vector2D {
public final double x;
public final double y;
public Vector2D(double angle) {
double angleRad = Math.toRadians(angle);
x = Math.cos(angleRad);
y = -Math.sin(angleRad);
}
public Vector2D(double x, double y) {
this.x = x;
this.y = y;
}
public Vector2D(Vector2D value) {
x = value.x;
y = value.y;
}
public Vector2D add(Vector2D v) {
return new Vector2D(x + v.x, y + v.y);
}
public int intX() {
return (int) Math.round(x);
}
public int intY() {
return (int) Math.round(y);
}
public boolean isInside(Rectangle rect) {
return rect.contains(new Point(intX(), intY()));
}
public Vector2D multiply(double d) {
return new Vector2D(x * d, y * d);
}
@Override
public String toString() {
return String.format("(%.2f, %.2f)", x, y);
}
}[/CODE]
[CODE lang="java" title="MyFloatDocument stellt Eingabe von Floatwerten sicher"]import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.PlainDocument;
@SuppressWarnings("serial")
public class MyFloatDocument extends PlainDocument {
public void insertString(final int offset, String text, final AttributeSet attributeSet) {
try {
if (!text.matches("[+-]?([0-9]*[.])?[0-9]+"))
return;
super.insertString(offset, text, attributeSet);
} catch (BadLocationException e) {
e.printStackTrace();
}
}
}[/CODE]