Hi Leute,
ich hab ein kleines Problem, weiß nicht wie ich bei einem Sudoku die einzelnen Zellen updaten soll.
Was ich bis jetzt hab:
Kann mir nicht vorstellen das ich hier am richtigen weg bin wäre nett wenn ihr mir vielleicht einen tipp geben könnt.
Angabe:
lg
ich hab ein kleines Problem, weiß nicht wie ich bei einem Sudoku die einzelnen Zellen updaten soll.
Was ich bis jetzt hab:
Java:
public class Cell {
public int size;
public int value;
public int[] options = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
/**
* Constructs a cell for a Sudoku of given size.
*
* @param size
* the size n of the Sudoku
* @param value
* the value of the cell, may be EMPTY
*
*/
public Cell(int size, int value) {
this.size = size;
this.value = value;
}
/**
* Returns the value of this cell.
*
* @return the value
*
*/
public int getValue() {
return this.value;
}
/**
* Indicates if cell is empty, i.e., has no value.
*
* @return true, if empty
*
*/
public boolean isEmpty() {
return this.value == 0;
}
/**
* Erases the given value from options and returns changes.
*
* @return true, if erase action changed options
*
*/
public boolean erase(int value) {
for (int i = 0; i < this.options.length; i++) {
if (this.value == options[i]) {
options[i] = 0;
return true;
}
}
return false;
}
/**
* Returns the current number of possible values of this cell.
*
* @return the number of possible values, 0, if the cell has a value
*
*/
public int getOptionCount() {
if (this.isEmpty()) {
int count = 0;
for (int i = 0; i < this.options.length; i++) {
if (this.options[i] != 0) {
count++;
}
}
return count;
}
return 0;
}
/**
* Checks options to give this cell a value and returns changes.
*
* @return true, if cell state changed from empty to not empty
*
*/
public boolean update() {
if(this.isEmpty()) {
for (int i = 0; i < this.options.length; i++) {
if (this.options[i] != 0) {
return true;
}
}
}
return false;
}
}
Kann mir nicht vorstellen das ich hier am richtigen weg bin wäre nett wenn ihr mir vielleicht einen tipp geben könnt.
Angabe:
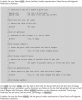
lg