Hallo zusammen,
Ich habe eine Aufgabe gegeben, wo ich unter anderem die Klasse Row implementieren soll:
Die Klasse Row stellt einen Eintrag (eine Zeile bzw. ein Tupel) einer Tabelle dar und hat die folgenden Instanzvariablen:
Dabei bekomme ich jetzt folgende Fehlermeldung:
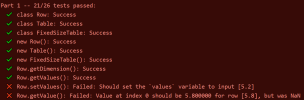
Bei dem setValues und getValue scheint es also Probleme zu geben - ich habe jetzt schon ziemlich viel herumprobiert, komme aber nicht wirklich auf meinen fehler. Kann mir da vielleicht jemend einen Tipp geben?
Schöne Weihnachtsfeiertage,
LG Euler
Ich habe eine Aufgabe gegeben, wo ich unter anderem die Klasse Row implementieren soll:
Die Klasse Row stellt einen Eintrag (eine Zeile bzw. ein Tupel) einer Tabelle dar und hat die folgenden Instanzvariablen:
- dimension: Die maximale Anzahl an Attributen (Spalten) eines Eintrags.
- values: Die Werte der jeweiligen Attribute als double-Array.
Java:
public class Row {
private int dimension;
private double[] values;
/**
* Creates a new row.
*
* All entries are initially set to `Double.NaN`.
*
* @param dimension The number of elements of that this row contains.
*/
public Row(int dimension) {
this.dimension = dimension;
this.values = new double[dimension];
for (int i = 0; i < dimension; i++) {
this.values[i] = Double.NaN;
}
}
/**
* Get the dimension of this row.
*/
public int getDimension() {
return dimension;
}
/**
* Get the values stored in this row.
*/
public double[] getValues() {
return values;
}
/**
* Sets the values of this row.
*
* This replaces the entire array, not just its elements, and updates
* the dimension of this row (according to the length of the given
* `double` array).
*
* @param values The `double` array that replaces the `double` array
* of the current row.
*/
public void setValues(double[] values) {
this.values = values.clone();
this.dimension = values.length;
}
/**
* Get a value in this row.
*
* @param index The index of the value to get.
* @return If the index is invalid (that is, negative or our of bounds),
* `Double.NaN`; otherwise, the value at that index (which may also be
* `Double.NaN`).
*/
public double getValue(int index) {
if (index < 0 || index >= dimension) {
return Double.NaN;
}
return values[index];
}
/**
* Set a value in this row.
*
* @param index The index of the value to set.
* @param value The value to set the element at that index to.
* @return `false` if the index is invalid (that is, negative or our of
* bounds), or if the element at that index already has the value `value`;
* `true` otherwise.
*/
public boolean setValue(int index, double value) {
if (index < 0 || index >= dimension || Double.isNaN(value)) {
return false;
}
if (values[index] == value) {
return false;
}
values[index] = value;
return true;
}
/**
* Get the average of all values in this row.
*
* @return The arithmetic mean of all non-NaN entries in this row; note that
* NaN entries are to be excluded entirely; e.g. if a row has 10 entries;
* only three of which—`a`,`b`, `c`—are not NaN, then this should return
* `(a + b + c) / 3`.
*/
public double getAverage() {
double sum = 0.0;
int count = 0;
for (double value : values) {
if (!Double.isNaN(value)) {
sum += value;
count++;
}
}
if (count == 0) {
return Double.NaN;
}
return sum / count;
}
/**
* Convert a row to a string.
*/
@Override
public String toString() {
StringBuilder s = new StringBuilder();
double[] vs = getValues();
s.append("|");
for (double v : vs) {
s.append(String.format(" %5.3f |", v));
}
s.append(String.format(" %5.3f |", getAverage()));
return s.toString();
}
}
Dabei bekomme ich jetzt folgende Fehlermeldung:
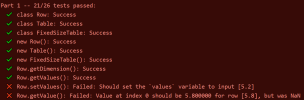
Bei dem setValues und getValue scheint es also Probleme zu geben - ich habe jetzt schon ziemlich viel herumprobiert, komme aber nicht wirklich auf meinen fehler. Kann mir da vielleicht jemend einen Tipp geben?
Schöne Weihnachtsfeiertage,
LG Euler